9. Module and class diagrams¶
A module diagram illustrates the module API. The API consists of the Requests received by the module and the Broadcasts transmitted by the module. A class diagram illustrates the properties (also called “attributes”, “data” or “state”) of the module and methods used to access the properties.
9.1. Base Module¶
All modules inherit from the Base Module. All modules implement the base Requests and Broadcasts defined below:
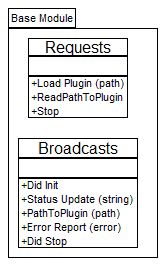
Figure 22: Base Module
9.1.1. Requests¶
Requests are transmitted to a module from the framework. They consist of a request type and a data type. The requests for the base module are as follows:
9.1.1.1. Load Plugin¶
The event module uses plugins to create different events. Plugins each include a mathematical function of time representing a power system event on a particular bus in the power system. Multiple buses are represented by instantiating multiple event modules with different event plug-ins for each instantiation. The Load Plugin request parameter is a path to an event plugin initialization (.ini) file.
9.1.1.2. Stop Request¶
Requests the module to stop and unload from memory.
9.1.2. Broadcasts¶
The framework and any other module or application can register to receive broadcasts from a module.
9.1.2.1. Did Init¶
Standard output for all modules. Confirms that the module has initialized
9.1.2.2. Status Update¶
Standard output for all modules. Sends a string informing the framework of some kind of change in the module’s status with the exception of errors which are transmitted vi the error report broadcast.
9.1.2.3. Error Report¶
Standard output for all modules. On the occurrence of an error in the module, broadcasts the error cluster.
9.1.2.4. Event Reports¶
Broadcasts an array of structures of ideal PMU data. The structure is as follows: * Bus Number (integer)
- UTC Timestamp (double)
- Synchrophasors ([complex double])
- Frequency (double)
- ROCOF (double)
The synchrophasors are a one-dimensional array of complex doubles, each element represents one phase of voltage or current from the bus represented by the event module.
9.2. Base Plugin Class¶
All plugin classes inherit from the base plugin class. The class contains no properties. The methods are as follows:
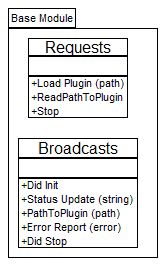
Figure 23: Base Plugin Class
9.2.1. Methods¶
9.2.1.1. Create / Destroy¶
Methods common to all base classes. Generally, does nothing and are not overridden unless something special must be done to create and destroy the objects at runtime.
9.2.1.2. Implementation Error¶
If a base class must be overridden by a child, this method ensures that any base class object that is not overridden throws an error.
9.2.1.3. GetPathToClass¶
Plugin children must exist on the hard drive of the system that they are being instantiated, this method allows a base class to lookup the path to the class file from the path contained in the child’s .ini file.
9.2.1.4. LoadClassFromFileOnDisk¶
Loads the child class from the file pointed to by the path in the child’s .ini file.
Event Module
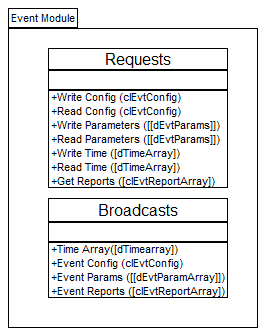
Figure 4: Event Module diagram
9.2.2. Requests¶
In addition to the requests inherited from the base module, the event module supports the following requests:
9.2.2.1. Write/Read Config¶
The event module configuration data consist of a clConfig structure containing the UTC T0 (time zero), the PMU reporting Rate (read only), and the PMU Nominal Frequency.
9.2.2.2. Write/Read Parameters¶
Event parameters are a two dimensional array of doubles. The meaning of the rows and columns of the array are dependent on the event plug-in and are specified in the plug-in’s .ini file.
9.2.2.3. Write/Read Time¶
Time is a vector of time offsets to UTC T0 for which to create the ideal PMU reports. The time vector is signed, meaning that reports from before T0 may be created. The read-only PMU reporting rate from the Config cluster is the mean of the differences between the times in the time vector.
9.2.2.4. Get Reports¶
Requests broadcast of an array of report clusters. For each element in the time vector, there will be one element in the array of report clusters with a timestamp of the UTC T0 plus the value of the time array element. For details of the report structure data see 6.1.2.4.
9.2.2.5. Stop Request¶
Requests the module to stop and unload from memory.
9.2.3. Broadcasts¶
In addition to the broadcasts inherited from the base module, the event module supports the following requests:
9.2.3.1. Time Array¶
Broadcasts the time vector array of doubles.
9.2.3.2. Event Config¶
Broadcasts the event configuration cluster (see 6.2.1.1).
9.2.3.3. Event Parameters¶
Broadcasts the two dimensional array of event parameters (see 6.2.1.2).
9.2.3.4. Did Stop¶
Standard output for all modules. Broadcast when the module stops and is about to unload from memory
9.2.4. Event Plugins¶
9.2.4.1. Event Plugin Base Class¶
end Users may create their own plugins. All event plugins inherit from the event plugin base class. Most of the methods allow for data to be written and read to the plugin. It is expected that the GetReports method will be overridden by each of the event type child classes to provide reports generated specifically by that event type
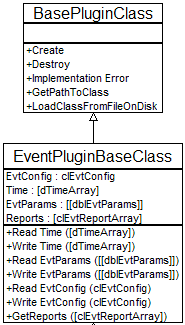
Figure 25: Event Plugin Base Class diagram
All event plugin child classes inherit from this base class. The methods have all been explained in 6.2.1 and 6.2.2
9.2.4.2. C37.118 Event Plugin¶
TODO:
9.2.4.3. Event From CSV Plugin¶
TODO:
9.2.4.4. IEEE Bus System Event¶
TODO:
9.2.4.5. Ringdown Event¶
TODO:
9.2.4.6. User Events¶
TODO:
9.3. PMU Impairment Module¶
Figure 26: PMU Impairment module
9.3.1. Requests¶
In addition to the requests inherited from the base module, the event module supports the following requests:
9.3.1.1. Write/Read ImpairConfig¶
The PMU Impairment module configuration data consists of a clImpairConfig structure. The elements are TBD at this time.
9.3.1.2. Write/Read ImpairParams¶
The impairment parameters are a 2 dimensional array of double that are defined by the impairment algorithm.
9.3.1.3. Write EvtReports¶
Event reports are generated by the Event module as a array of data structure clReports. For details of the report structure see 6.1.2.4
9.3.1.4. GetImpairReports¶
Requests an array of PMI Impairment reports in the clReports structure format. For details of the report structure see 6.1.2.4
9.3.1.5. Broadcasts¶
In addition to the broadcasts inherited from the base module, the event module supports the following requests:
9.3.1.6. ImpairConfig¶
Response to the Read ImpairConfig Request. Broadcasts the module’s clImpairConfig data structure.
9.3.1.7. ImpairParams¶
Response to the Read ImpairParam Request. Broadcasts the module’s clImpairParam two dimensional array of doubles.
9.3.1.8. ImpairReports¶
Response to the getImpairReports Request. Broadcasts an array of impaired PMU data reports in the clReports structure format. For details of the report structure see 6.1.2.4
9.4. PMU Impairment class¶
Figure 27: PMU Impairment class
A child of the BasePluginClass. The methods are all described in the preceding sections:
9.5. Network Impairment Module¶
Figure 28: Network Impairment Module diagram
9.5.1. Requests¶
In addition to the requests inherited from the base module, the event module supports the following requests:
9.5.1.1. Write TimeArray¶
TimeArray is a vector of time offsets to UTC T0 which were used to create the ideal PMU reports. This time vector is the ideal time vector for which the PMU reports would have been transmitted from the PMU.
9.5.1.2. Read/Write ImpParams¶
A two dimensional array of doubles make up the network impairment parameters.
9.5.1.3. Read/Write LossThreshold¶
The one dimensional array of doubles defines the loss threshold.
9.5.1.4. GetNetTime¶
Requests that the Net Time array be broadcast. The Net Time is an impaired version of the time vector.
9.5.1.5. GetPktLoss¶
Requests that the Packet Loss Data be broadcast.
9.5.2. Broadcasts¶
In addition to the broadcasts inherited from the base module, the event module supports the following requests:
9.5.2.1. ImpParams¶
Response to Read ImpParams request. Broadcasts the two dimensional array of doubles that are the network impairment parameters.
9.5.2.2. LossThreshold¶
Response to Read LossThreshold request. Broadcasts the one dimensional array of doubles that are the network Loss Threshold.
9.5.2.3. NetTime¶
Response to GetNetTime. Broadcasts the one dimensional array of doubles which ate the Net Time vector (an impaired version of the Time Array input to the event module.
9.5.2.4. PktLoss¶
Response to GetPktLoss. Broadcasts the two dimensional array of doubles which are the Packet Loss data.
9.6. Network Impairment class¶
Figure 29: Network Impairment Class diagram
The properties and methods for the Network Impairment class have all been described in 6.4.1 and 6.4.2.
9.7. Flag Impairment Module¶
PMU reports contain flags which carry information about the data contained in the PMU report. This module simulates the settings of those flags similar to the flags found in typical and atypical PMU streams.
Figure 30: Flag Impairment Module
9.7.1. Requests¶
9.7.1.1. Write FlagImpairConfig¶
A cluster of configuration information for the FlagImpairment module
9.7.1.2. Write FlagImpairParams¶
A one dimensional array of doubles setting the parameters for the flag impairment.
9.7.1.3. Write TimeArray¶
The one dimensional array of times from the Event module
9.7.1.4. GetFlagImpairment¶
Requests broadcast of the one dimensional array of 32-bit unsigned integers which represent the impaired status flags
9.8. Flag Impairment Plugin class¶
This class is a pluggable class. The two child classes will be the C37.118 Flag Impairment class and the IEC 61850 Flag Impairment class.
Figure 31: Flag Impairment Plugin Class
The attributes and methods of the FlagImpairment class have all been described in 6.5
9.9. Output to File Module¶
The module receives ideal PMU reports from the event module, impaired PMU reports from the PMU Impairment module and Network Time and Packet Loss data from the Network Impairment module and prepares the data for output to a file format determined by the plug-in for the module.
Figure A‑32: Output to File Module
9.9.1. Requests¶
In addition to the requests inherited from the base module, the event module supports the following requests:
9.9.1.1. Write OutputToFilePluginIniPath¶
Writes the path to the plugin’s .ini file and loads the plugin.
9.9.1.2. Read OutputToFilePluginIniPath¶
Reads the path to the plugin’s .ini file.
9.9.1.3. Write/Read OutputPath¶
The top level path to where the output files will be placed.
9.9.1.4. Write/Read ConfigOptions¶
This is the configuration of the PMU which will be used in the output file
9.9.1.5. Write NetTime¶
A one dimensional array of doubles generated by the network impairment module is an impaired version of the time vector used to generate the ideal and impaired PMU reports.
9.9.1.6. Write PktLoss¶
A one dimensional array of doubles generated by the Network Impairment module used to mark data written to the output file as lost.
9.9.1.7. Write FlagImpairArray¶
A one dimensional array of Int32 representing the PMU flags from the Flag Impairment Module.
9.9.1.8. OutputReferenceFile¶
Requests the module to write the file of reference (ideal) output data in the format determined by the plugin.
9.9.1.9. OutputImpairedFile¶
Requests the module to write the file of impaired output data in the format determined by the plugin.
9.9.1.10. OutputErrorData¶
Requests the module to write the error data (TVE, ME, PE, FE, RFE) for output to an error file.
9.9.2. Broadcasts¶
In addition to the requests inherited from the base module, the broadcasts are:
9.9.2.1. OutputToFilePluginIniPath¶
Path to the plugin’s .ini file
9.9.2.2. OutputPath¶
Path to the top level directory where the output files will be written
9.9.2.3. ConfigOptions¶
The cluster of PMU configuration options
9.9.2.4. Output to File base plugin class¶
A plugin class where the children of the class each output to a different file format. Example file formats are C37.118.2 csv, WECC-JSIS, and Comtrade.
Figure A‑33: Output to File class
The properties and methods for the Network Impairment class have all been described in 6.6.1